本文共 1917 字,大约阅读时间需要 6 分钟。
基于TI TMS320DM6467T无操作系统Camera Link智能图像分析平台 |
| 1、板卡概述 该板卡是我公司推出的一款具有 高可靠性、效率 大化、无操作系统的智能视频处理卡,是机器视觉开发上的 选。 它集成 ARM9和 C64x+ DSP内核,性能比普通 DaVinci处理器提升数倍。 DM6467能以十倍的性能和十分之一的价格同时进行多种格式 HD编码转换。其内置高清视频协处理器( HD-VICP),特别适用于高清视频处理。带有 H.264 X-HD编解码器,同时支持多路 H.264实时编码,轻松应用于各种视频处理领域。支持 1080P@30fps/1080i/720P@60fps视频编解码。 该系统是由两块核心芯片组成,分别是TI DSP TMS320DM6467 DaVinci处理器和Altera FPGA EP3C40F484C8的芯片。以TI的DSP TMS320DM6467 DaVinci处理器作为主芯片,可用于高速数据、视频信号检测,分析等应用;FPGA处理芯片为EP3C40F484C8,兼容EP3C16F484C8的设计,支持Camera Link的数据输入和DVI的输出,主要用于处理高清图像输入信号,进行智能分析。 该系统同时包含FPGA视频增强处理器,大大提高了对高清视频的处理速度,具有 强的嵌入式视频处理能力。支持视频H.264,JPEG压缩,支持车牌识别,人脸识别等算法开发,可进行运动检测,火灾检测,交通监控,智能行为识别,行人监测,可疑人员追踪,行人计数,可疑静止物体监测等。 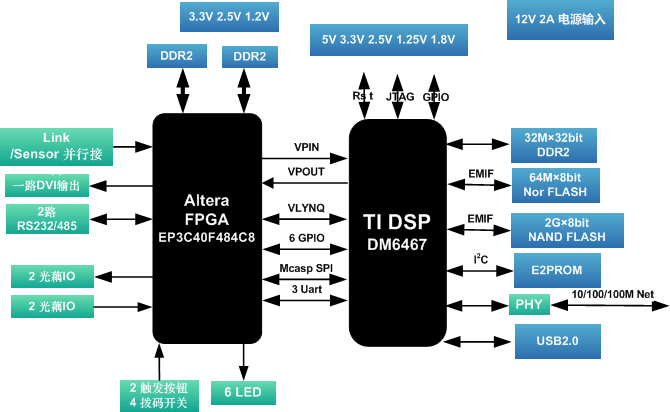 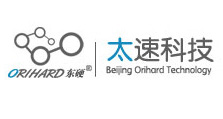 2、板卡性能介绍 一、DSP部分: (1) DSP芯片性能: DM6467实现了在各种视频终端产品间的无缝内容传输。它集成了ARM926EJ-S内核与600MHz C64x+ DSP内核,并采用高清视频/影像协处理器(HD-VICP)、视频数据转换引擎以及目标视频端口接口。在执行高达H.264 HP@ L4(1080p 30fps、1080i 60fps、720p 60fps)的同步多格式高清编码、解码与转码方面,实现了 过3GHz的DSP处理能力。主要针对媒体网关、多点控制设备、数字媒体适配器、视频安全监控DVR以及IP机顶盒等应用。 (2) 与FPGA互连接口: (a)VPIN:视频输入。支持1080P 30fs 彩色图像输入。 (b)VPOUT:视频输出。支持1080p 60fs 彩色图像输出。 (c)GPIO:通用可编程输入输出口。其实就是一些裸的接口,再接口上加高电平,然后就可以在软件里面读取到,还会产生中断。 (d)Uart:3通路接口。 二、FPGA部分: (1)根据本系统的运算性能和外设I/O的需求,FPGA卡选用CycloneIII系列的EP3C40F484C8芯片,它具有39600个逻辑单元、2340Kbit存储单元,126个乘法器、4个PLL, 331个外部独立I/O, 高运行时钟高达300MHz 。该性能已经满足本系统的要求。 (2) FPGA图像缓存SDRAM: 选用两片大容量的SDRAM,MT48LC16M16A2 (4 Meg x 16 x 4 banks)独立挂接在FPGA上,可以构成乒乓操作,也可以组合成大存储空间。 (3)接口介绍: (a) FPGA外接2路RS232串口, (b) FPGA外接 一路Camera Link输入,Camera Link 支持Base 模式 (c) FPGA外接1路DVI输出,支持1080P 60fs模式。 3、软件功能: 一、DSP软件功能: (1) 支持千兆网络传输程序,支持ping,TCP、UDP、IP传输协议。 (2) 支持H.264 JPEG 压缩编码,支持动态目标识别。 (3) 支持1K字节的引导,Flash 烧写测试程序。 (4) DSP软件支持无操作系统控制,直接利用BIOS 完成ARM,DSP,编解码核的控制传输使用。 二、FPGA软件功能: (1)支持FPGA程序JTAG,AS模式配置。 (2)支持FPGA的CameraLink图像输入,RS232/485信号传输 (3)支持FPGA的 SDRAM数据读写。 4、物理特性 130mm (L) × 75mm (l) × 20mm (h) 温度:0 到 +50 °C 湿度:10~90% 非凝结性 5、供电要求 输入:12V直流,10W 直径3.5毫米。电源插座连接器 电源电压和复位管理 瞬态电压抑制器和EMI滤波器 6、应用范围 机器视觉,多路视频编码、高清视频编解码等。 | | |
转载地址:http://tunlz.baihongyu.com/